5.2.1 列表框
常用列表框属性:
常用列表框方法:
例 7 :将文本框改为列表框 (list) 求 1000 到 1100 之间的所有素数。
程序代码:
Private Sub Command1_Click()
List1.Clear
For n = 1001 To 1100 Step 2
s = 0
For i = 2 To Int(Sqr(n))
If n Mod i = 0 Then
s = 1: Exit For
End If
Next
If s = 0 Then List1.AddItem n
Next
End Sub
执行结果:
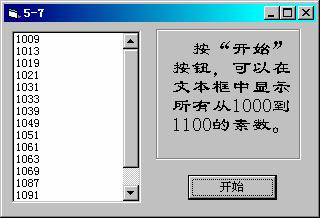
例 8 :找出 1-9999 之间的同构数。
程序代码:
Private Sub Command1_Click()
List1.Clear
For n = 1 To 9999
Select Case n
Case n ^ 2 Mod 10
List1.AddItem Format(n, "@@@@@") & Format(n ^ 2, "@@@@@@@@@@")
Case n ^ 2 Mod 100
List1.AddItem Format(n, "@@@@@") & Format(n ^ 2, "@@@@@@@@@@")
Case n ^ 2 Mod 1000
List1.AddItem Format(n, "@@@@@") & Format(n ^ 2, "@@@@@@@@@@")
Case n ^ 2 Mod 10000
List1.AddItem Format(n, "@@@@@") & Format(n ^ 2, "@@@@@@@@@@")
End Select
Next
End Sub
执行结果:
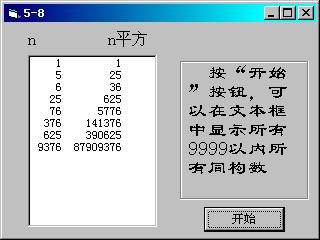
例 9 :小学生做加减法的算术练习程序。
程序代码:
Private Sub Command1_Click()
Unload Me
End Sub
Private Sub Form_Activate()
Randomize (Time)
a = Int(10 + 90 * Rnd)
b = Int(10 + 90 * Rnd)
p = Int(2 * Rnd)
Select Case p
Case 0
Label1.Caption = a & " + " & b & "="
Text1.Tag = a + b
Case 1
If a > b Then t = a: a = b: b = t
Label1.Caption = a & "-" & b & "="
Text1.Tag = a - b
End Select
Form1.Tag = Val(Form1.Tag) + 1
Text1.SelStart = 0
Text1.Text = ""
End Sub
Private Sub Text1_KeyPress(KeyAscii As Integer)
If KeyAscii = 13 Then
fm = "!@@@@@@@@@@@@@@"
If Val(Text1.Text) = Text1.Tag Then
Item = Format(Label1.Caption & Text1.Text, fm) & " √ "
List1.Tag = Val(List1.Tag) + 1
Else
Item = Format(Label1.Caption & Text1.Text, fm) & " × "
End If
List1.AddItem Item, 0
Label2.Caption = " 共 " & Form1.Tag & " 题 ," & Chr(13) & " 正确率为: "
Label3.Caption = Format(Val(List1.Tag) / Val(Form1.Tag), "#0.0#%")
Form_Activate
End If
End Sub
执行结果:
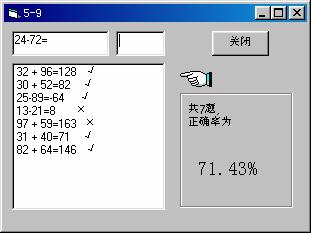
例 10 :利用循环结构和列表框控件,设计的“选项移动”窗口。
程序代码:
Private Sub Command1_Click()
i = 0
Do While i < List1.ListCount - 1
If List1.Selected(i) = True Then
List2.AddItem List1.List(i)
List1.RemoveItem i
Else
i = i + 1
End If
Loop
End Sub
Private Sub Command2_Click()
For i = 0 To List1.ListCount - 1
List2.AddItem List1.List(i)
Next
List1.Clear
End Sub
Private Sub Command3_Click()
i = 0
Do While i < List2.ListCount
If List2.Selected(i) = True Then
List1.AddItem List2.List(i)
List2.RemoveItem i
Else
i = i + 1
End If
Loop
End Sub
Private Sub Command4_Click()
For i = 0 To List2.ListCount - 1
List1.AddItem List2.List(i)
Next
List2.Clear
End Sub
Private Sub Form_Load()
List1.AddItem " 电冰箱 "
List1.AddItem " 洗衣机 "
List1.AddItem " 彩色电视机 "
List1.AddItem " 组合音箱 "
List1.AddItem " 影碟机 "
List1.AddItem " 电水壶 "
List1.AddItem " 饮水机 "
List1.AddItem " 微波炉 "
List1.AddItem " 照相机 "
End Sub
执行结果:
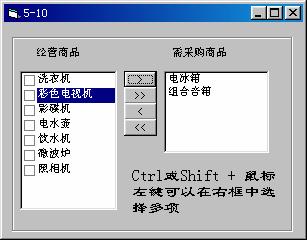 5.2.2 组合框
组合框兼有 TextBox( 文本框 ) 和 ListBox( 列表框 ) 两者的功能,用户可以通过输入文本或选择列表中的项目来进行选择。
有三种形式的组合框:
下拉列表框 (Style 属性值为 2) :和列表框一样,为用户提供了一些选项和信息 的列表。在列表框中,任何时候都能看到多个选项,而在下拉列表框中,一般只能看到一个选项。
简单组合框 (Style 属性值为 1) :将文本框和列表框简单地组合在一起 , 上面为可供输入的文本框 , 下面为普通的列表框。
下拉组合框 (Style 属性值为 0) :将文本框和下拉列表框合在一起,用户可以单击向下按钮来显示可滚动的选项列表,还可以输入列表中所没有的新选项。
例 11 :将例 9 算术练习中的列表框改为组合框。
程序代码:
Private Sub Command1_Click()
Unload Me
End Sub
Private Sub Command2_Click()
Form10.Tag = 0#
Combo1.Tag = 0#
Combo1.Clear
Label2.Caption = ""
Form_Activate
Text1.SetFocus
End Sub
Private Sub Form_Activate()
Randomize (Time)
a = Int(10 + 90 * Rnd)
b = Int(10 + 90 * Rnd)
p = Int(2 * Rnd)
Select Case p
Case 0
Label1.Caption = a & " + " & b & "="
Text1.Tag = a + b
Case 1
If a > b Then t = a: a = b: b = t
Label1.Caption = a & "-" & b & "="
Text1.Tag = a - b
End Select
Form10.Tag = Val(Form10.Tag) + 1
Text1.SelStart = 0
Text1.Text = ""
End Sub
Private Sub Text1_KeyPress(KeyAscii As Integer)
If KeyAscii = 13 Then
fm = "!@@@@@@@@@@@@@@"
If Val(Text1.Text) = Text1.Tag Then
Item = Format(Label1.Caption & Text1.Text, fm) & " √ "
Combo1.Tag = Val(Combo1.Tag) + 1
Else
Item = Format(Label1.Caption & Text1.Text, fm) & " × "
End If
Combo1.AddItem Item, 0
Combo1.ListIndex = 0
Label2.Caption = " 共 " & Form10.Tag & " 题 ," & Chr(13) & " 正确率为: "
Label3.Caption = Format(Val(Combo1.Tag) / Val(Form10.Tag), "#0.0#%")
Form_Activate
End If
End Sub
执行结果:
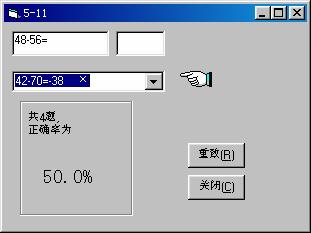
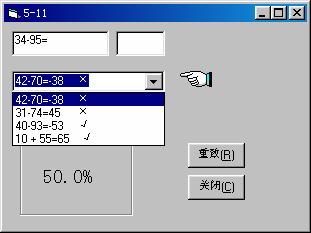
例 12 :“简易抽奖机”。在组合框中输入号码,按“开始”按钮可以得到中奖的号码。
程序代码:
Private Sub Combo1_KeyPress(KeyAscii As Integer)
If KeyAscii = 13 Then
List1.AddItem Combo1.Text, 0
End If
If KeyAscii = 27 Then
If List1.ListIndex <> -1 Then
List1.RemoveItem Combo1.ListIndex
End If
End If
End Sub
Private Sub Command1_Click()
Randomize
n = List1.ListCount
a = Int(Rnd * n)
List1.ListIndex = a
MsgBox " 中奖号码是: " & Chr(13) & List1.Text, 0, " 恭喜! "
If Text1.Text = List1.Text Then
MsgBox " 你中奖了!! ", 48, " 恭喜发财!!! "
End If
End Sub
执行结果:
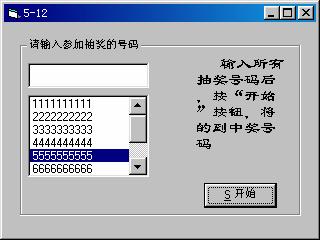
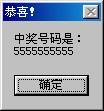
例 13 :在例 12 中使用下拉组合框,可能更加节省空间。
程序代码:
Private Sub Combo1_KeyPress(KeyAscii As Integer)
If KeyAscii = 13 Then
Combo1.AddItem Combo1.Text, 0
Combo1.SelStart = 0
Combo1.SelLength = Len(Combo1.Text)
Text1.Text = Combo1.ListCount
End If
If KeyAscii = 27 Then
If Combo1.ListIndex <> -1 Then
Combo1.RemoveItem Combo1.ListIndex
Text1.Text = Combo1.ListCount
End If
End If
End Sub
Private Sub Command1_Click()
Randomize
n = Combo1.ListCount
a = Int(Rnd * n)
Combo1.ListIndex = a
Label1.Caption = " 中奖号码是: " & Combo1.Text
End Sub
执行结果:
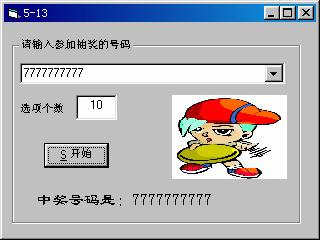
|